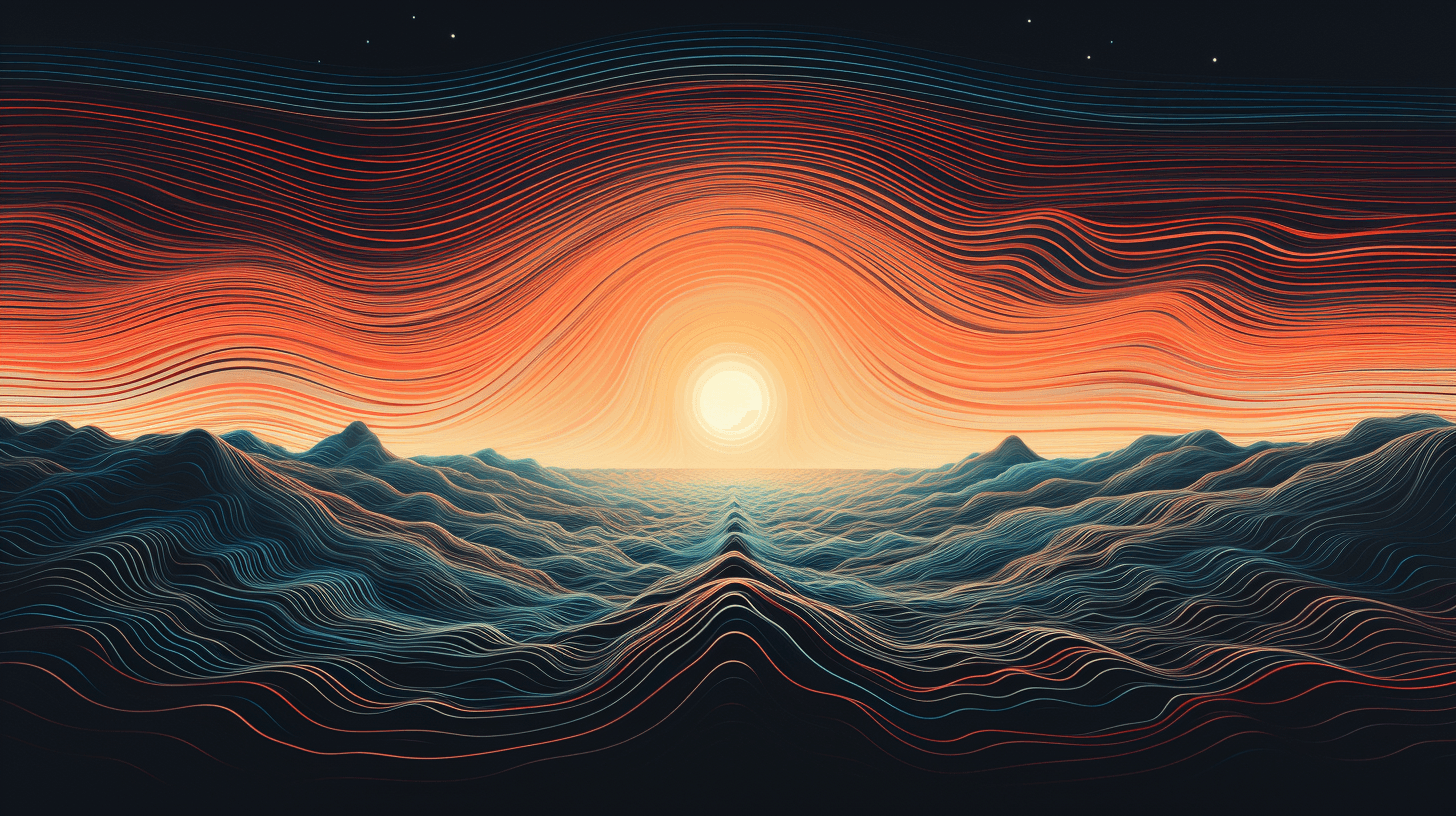
Swift: A Language for Modern Development
Swift is a general-purpose, multi-paradigm and compiled language for iOS, macOS, watchOS, tvOS, and Linux applications. Swift is the outcome of the most recent research on programming languages and created by team led by Chris Lattner at Apple.
Cocoa programming had always been done mostly in Objective-C, first on Mac OS and then on iOS until that point. The Cocoa frameworks that give an iOS app its functionality are based on Objective-C, they expect to be spoken to in Objective-C. For all its flaws, Objective-C was the language we learned to live with as the price of Cocoa programming.
Is it possible to communicate with Cocoa in a different language? This is where possibilities become reality, the answer is yes. Swift is explicitly designed to interface with most of the Cocoa APIs.
Swift is easy to learn and easy to implement, even if you’re a new programmer. Some of the key features:
- OpenSource: Swift’s founders recognized that to create a defining language, the technology needed to be accessible to everyone. With this decision, Swift language gained a large community and a wide range of open source tools.
- Object-orientation: Swift is a modern, object-oriented language. It is purely object-oriented:
Everything is an object
. - Safety: Swift uses a strong, static type system to ensure that types are correctly matched at compile-time, reducing runtime errors.
- Memory Managemen: Swift manages memory by using Automatic Reference Counting (
ARC
), which removes typical memory management mistakes and improve efficiency. - Modern Syntax: Swift's syntax is consistent, clear, and expressive, allowing developers to write code that is easy to read and maintain.
- Optimized Compiler: LLVM-based compiler optimizations enable Swift code to be compiled into highly efficient machine code.
Stable ABI and Backward Compatibility
In March 2019, Swift 5.0 was officially released. It introduced a stable version of the application binary interface (ABI) across Apple’s platforms.
ABI is an interface between two binary program modules. It is a collection of rules that allow applications and program parts, such as libraries or frameworks, to speak the same "binary language" and interact with one another. Typically, the ABI includes the following topics:
- Calling convention (how to call functions, pass arguments, return values)
- How data is represented in memory
- Processor instruction set, stack organization
- How an application should make system calls to the operating system
You write Swift code and want to share it with many apps. You make a framework and use the Swift 3.0 compiler. Then Apple brings out Swift 4.0. You're excited and make a new app with Swift 4.0 compiler. The problem is that your project requires certain functionalities from your previous framework. Is it going to work with new project? Nope. Before
ABI stability
both pieces of code had to be compiled with the same compiler for them to communicate. So, if your framework was compiled with Swift 3.0 and your app with Swift 4.0, they wouldn't work together. Now, with "ABI stability," things have changed. A framework compiled with Swift 5.0 can communicate with an app compiled with Swift 5.1.
Before stable ABI, the complete Swift standard library and runtime were included in every Swift app that was created. This was because Swift was evolving rapidly, making it impractical to include its fundamentals in iOS releases. ABI stability refers to the ability of a language to be packaged and linked directly into the operating system.
Swift's system footprint will decrease once it becomes an ABI stable language because apps won't need to package with swift dynamic libraries, and you'll still be able to take advantage of the OS's internal optimizations. Another advantage of stable ABI is developers will be able to write closed-source third-party libraries and release them as pre-compiled frameworks.
Swift in Action: Across Different Areas
Swift is adaptable and works well in a variety of programming scenarios, including server-side development, scripting, automation, and mobile app development. Due to its high performance, wide feature set, and clear syntax, it is becoming more and more popular among developers in different fields.
Swift in mobile app development
When developing apps for the iOS, iPadOS, watchOS, and tvOS platforms, Swift is mostly used. The following benefits highlight some advantages that developers can leverage while creating mobile applications with Swift:
- Swift has ability to integrate with Apple's SDKs and frameworks such as UIKit, AppKit, and SwiftUI.
- Clear and concise syntax makes your code more readable and maintainable.
- If additional developers are required, Swift lets you add them to your team easily, because the codebase is clear and simple, onboarding happens rather quickly.
- Safety features take center stage in Swift, such as optionals and type safety, helps developers write code that is less prone to runtime errors and vulnerabilities. This reduces the risk of crashes and security breaches in mobile apps, providing users with a more reliable and secure experience.
Swift in server-side development
Swift has gained interest in server-side development due to its open-source status and high-performance advantages. Swift can be used by developers to build RESTful APIs and web applications by utilizing server-side Swift frameworks such as Kitura, Vapor, and Perfect. These frameworks enable the following capabilities:
- Developing and deploying high-performance REST APIs for various client applications, including mobile apps and web apps.
- Creating web applications with server-side capabilities such as database access, authentication, file management, and more.
- Designing reusable server-side libraries that the Swift community can use or distribute across several projects.
Swift has a number of advantages for server-side development, including:
- Swift has impressive concurrency and performance support, enabling software developers to design scalable APIs and web applications.
- Safety features and error handling, improving the reliability and robustness of server-side code.
- The capacity to share code between client and server applications, which speeds up development and eliminates redundant code.
Swift for scripting and automation tasks
Swift is useful not only for server-side and mobile app development but also for scripting and automation tasks. Swift is a compiled language, which means that you typically need to compile your code into a binary before it can be executed. However, if you're familiar with Unix shells like Bash or Zsh, you know that scripts written in these shells can be run directly without the need for compilation. This is where Swift scripting comes in. By leveraging Swift's interactive shell, known as the REPL (Read-Eval-Print Loop), you can write and run Swift scripts without the need for compilation. This opens up a whole new world of possibilities for automating tasks and manipulating data using Swift.
Some common use cases for Swift scripting include:
- Creating and maintaining templates or configuration files.
- Manipulating data, such as transforming JSON or CSV files.
- Implementing batch procedures to big data sets.
Final Thoughts
Swift is more than just a coding language, it's a transformative instrument empowering developers. It helps us to learn new programing paradigms and enhance our ability to design efficient and scalable solutions. Swift has changed a lot since it started, and each update has made it better for developers. Ultimately, Swift's evolution translates to a more enjoyable and productive coding experience, empowering us to build more reliable applications with ease.