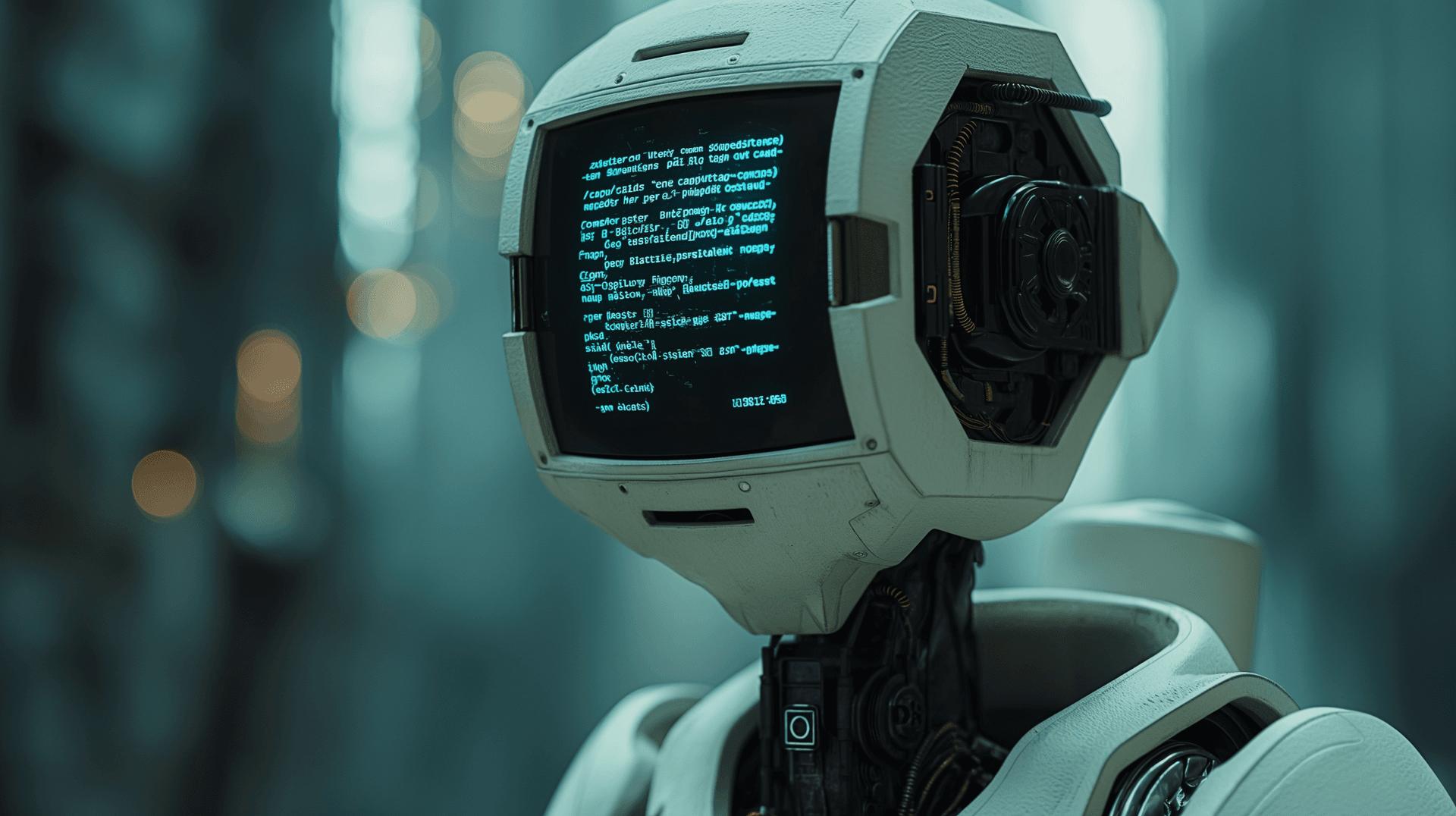
Swift Server-Side: Breaking Boundaries Beyond iOS
Swift is a general-purpose programming language that has been widely used for client-side development in Apple-specific hardware environments. The contribution of the open-source developer community has extended Swift to the other platforms(Linux, Windows), hence establishing Swift as a cross-platform programming language.
Expanding Boundaries
The fundamental difference between Client-side and Server-side Swift projects is their targeted platforms. Client-side Swift apps are only designed to run on Apple-hardware and OS’s. All client-side apps are exclusively designed for Apple products. This means that all the code we've written, along with any frameworks or libraries we've used, is compiled directly for the appropriate Apple hardware platforms.
The Server-side Swift world is much bigger. It's not just about macOS anymore; it also involves Ubuntu Linux, multiple cloud platforms, and Docker. With this expanded landscape comes the need for major architectural adjustments and a shift in project perspective. Specifically, you have to use tools and create frameworks that can function across all supported environments.
Adding Apple platform-compiled binaries to a server-side project using Carthage or CocoaPods ensures their compatibility only if our server is running on macOS. However, attempting to deploy the project to Linux or other platforms with these dependencies will result in a project build failure.
CocoaPods and Carthage command line tools are restricted to macOS, targeting hardware specific to Apple. At this step Swift Package Manager(SPM) comes to the stage as part of open-source Swift project.
Opening Up Swift and Its Components
The official version of open source Swift was first launched in December 2015. Since then, with increased community support and development, open source Swift has continuously gained popularity and maturity. The effort of the open source developer community is coordinated through the Swift programming language evolution process.
The Swift evolution process manages how new ideas are accepted, sets goals for future updates. It ensures Swift's robust evolution while maintaining application binary interface (ABI) stability, ensuring binary compatibility across Swift versions. Open source Swift includes Swift compiler, standard library, package manager, core libraries, test framework, and REPL/debugger. The following diagram shows the main components in open source Swift:
Swift standard library and Foundation
The Swift standard library is a Swift-only library which contains fundamental data types (like Int and Optional), protocols (like Hashable and Codable) and collections(like Array and Dictionaries).
Every Swift file automatically imports the standard library(import Swift
). It is open source.
Apple Foundation framework includes many features like file system access, data storage, string handling, networking, and localization functionality that are shared by all applications but are not part of the Swift standard library and language. Also, Foundation helps keep the Swift Standard Library focused and compact. In short, without Foundation, Swift wouldn't be the language we love.
Apple Foundation is closed source and designed by a team at Apple with Objective-C. Given its closed-source nature, the question arises: How can we utilize these features in our server-side applications? A team of individuals who are behind-the-scenes leaders worked together to create a big part of Foundation. This became Corelibs Foundation Project. Corelibs Foundation is a non-Objective-C reimplementation of Foundation for non-Apple platforms, particularly Linux. It mirrors Apple Foundation's designs as closely as possible. It's a good practice to check the project's Implementation status page periodically.
Backend Frameworks
We have explored the mindset behind server-side Swift and how it became achievable after Apple open-sourced it. It seems that we now have a very foundational toolkit for developing our backend applications as Swift developers. However, the question remains: Is this toolkit sufficient for constructing a fully-featured backend application before our hair begins to gray. Back-end frameworks like Vapor are essential because they provide a structured and efficient way to develop server-side applications.
They abstract away much of the low-level networking and infrastructure concerns, allowing developers to focus on building features and functionality. Vapor is an open-source web framework written in Swift, which allow us to write Web apps, and HTTP servers in Swift. Here's what Vapor is used for and why back-end frameworks like Vapor are necessary:
- Creating APIs: Vapor is commonly used for creating APIs for web and mobile applications. APIs allow different software systems to communicate with each other, enabling data exchange.
- Routing: Vapor provides a routing system that maps incoming HTTP requests to the appropriate handlers, making it easy to define endpoints for handling different types of requests.
- Middleware: Middleware in Vapor allows developers to add authentication, logging, error handling, and request/response modification to their applications in a modular and reusable way.
- Database Integration: Vapor integrates with various databases, including PostgreSQL, MySQL, SQLite, and MongoDB, allowing developers to store and retrieve data from their applications.
- Security: Vapor includes features for implementing security best practices such as input validation, encryption, and protection against common web vulnerabilities like cross-site scripting (XSS) and SQL injection.