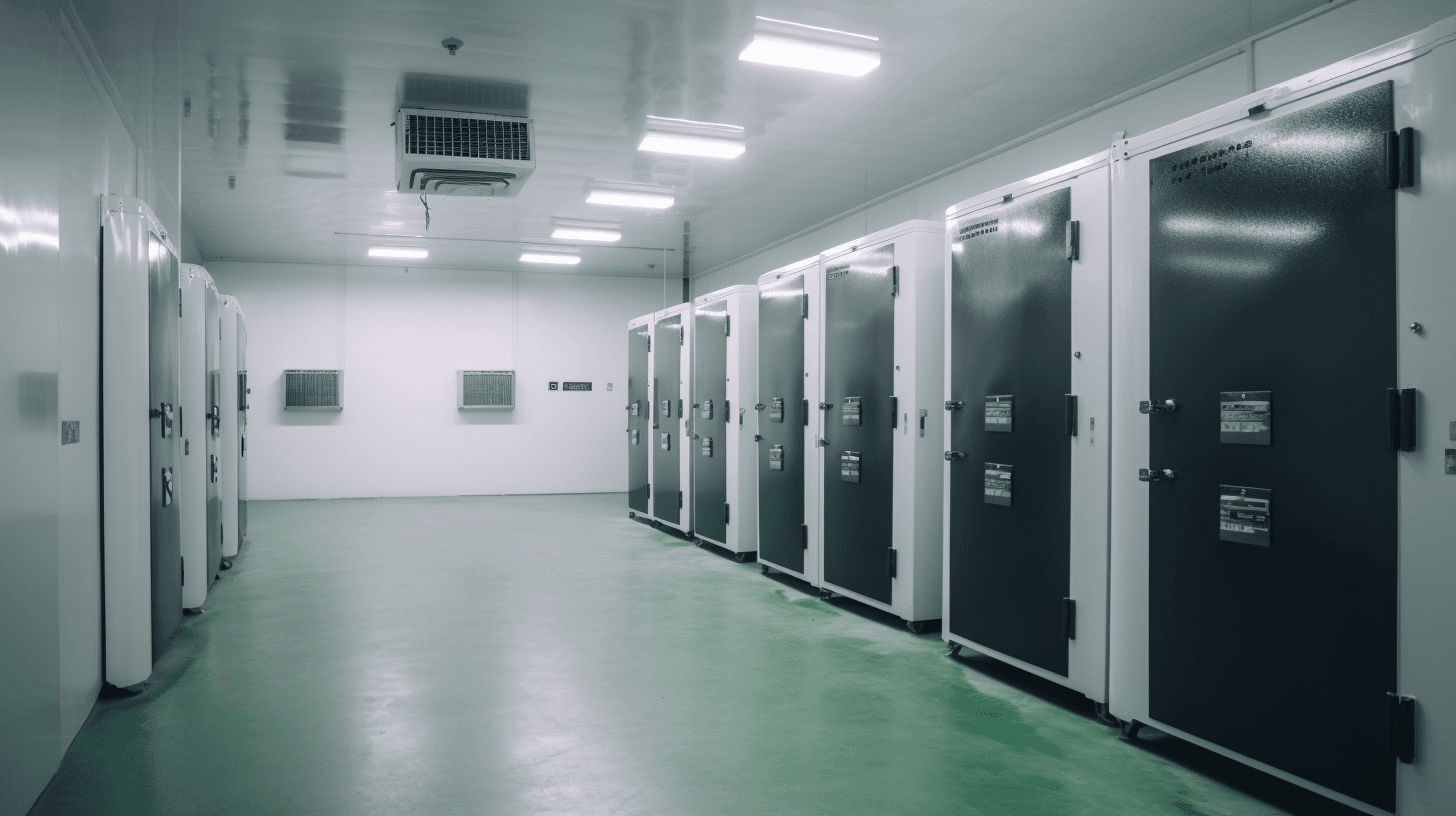
Understanding the Power of Application Sandboxing in iOS
Application sandboxing is a critical aspect of iOS security. It refers to the practice of isolating an app and its associated data and resources within a secure and controlled environment. An iOS user can install multiple apps on a single device. Therefore, the iOS platform ensures that these apps cannot interfere with each other in terms of memory usage and data storage.
So what is the effect of that on developers? And why does the operating system sandbox applications?
File System Security
Security is crucial for any modern application, and Apple offers a variety of tools for this purpose. For files, the iOS ecosystem includes three main security features:
- Sandboxing: The primary purpose of sandboxing is to enhance security by isolating apps and preventing them from interfering with each other or accessing unauthorized data. By confining each app to its own sandbox, iOS ensures that even if one app is compromised, it cannot compromise other apps or the underlying operating system. Sandboxing also helps to protect user privacy by limiting access to personal and sensitive data.
- Access control: File and directory access is controlled by access control lists (ACLs), providing detailed specifications on permissible actions and users authorized to perform them.
- Encryption: Thanks to iOS's data protection feature, you can secure the files in your app and prevent unauthorized access to them. When a user activates a passcode on their mobile device, privacy is activated automatically. The system encrypts and decrypts your content in the background while you read and write to your files as usual. This process is automatic and hardware accelerated.
_10do {_10 try data.write(to: fileURL, options: .completeFileProtection)_10}_10catch {_10 // Handle errors._10}
Why Is Sandboxing Essential?
iOS and other Apple platforms are based on a version of Unix. A Unix program has the same permissions and privileges as the user who is running it. This made sense in the past because users had to code and load your own programs. But these days, we use third-party apps which we did not write ourselves.
For Security reasons, Our apps should not have the same access as we do.
A malicious app or innocent bug could cause havoc on our system.
Therefore, we need to separate user’s privileges from third-party apps.
Apple solve this issue with a strict separation between apps and the operating system.
The outcome is App Sandbox
.
Introducing App Sandbox
The host device offers functionalities such as the calendar or camera that an app might want to use. To use them, the app needs to validate its identity and request permission from the OS and the user.
There are different types of resources that need authorization.
- Hardware: Microphone, Camera, sensors.
- Data from other apps: Photos, email, calendar and contact.
- User files: Files from the file system or the files app.
- Special Functionality: Push notifications, HomeKit access.
Sandboxing and Provisioning Profile Relation
At runtime, a sandboxed app might attempt a restricted operation such as accessing the camera. Operating system intercepts this operation and makes number of checks to let an app complete a restricted operation. The operating system asks questions, and a code-signed provisioning profile answers them. These are the questions and how your app answers them.
- Who are you? ->
app id
,team id
- Can I trust you? ->
certificate
- What do you want to do? ->
entitlements
Provisioning profile is file embedded in the app’s binary. When you want to deploy your app to a real device, you probably faced with this code signing process. Code signing your app ensures that it comes from a known source and has not been modified since it was last signed.
If we don’t have look at this process, sandboxing and provisioning might be easily seen as a collection of unrelated tasks.
How Can I Find the Sandbox?
In order to ensure that your files are being saved as you expected, you may find it helpful to take a peek inside the app's sandbox during development.
In the AppDelegate class, update the application(_:didFinishLaunchingWithOptions:)
method as shown below.
_10func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplicationLaunchOptionsKey: Any]?) -> Bool {_10 print(NSHomeDirectory())_10_10 return true_10}
When executed within the iOS Simulator environment, the path will take this form:
_10/Users/<user_name>/Library/Developer/CoreSimulator/Devices/<device_id>/data/_10Containers/Data/Application/<app_id>
When executed on a physical iOS device, the path:
_10/var/mobile/Containers/Data/Application/<app_id>
Copy that value from the console, switch to the Finder, choose Go → Go to Folder, paste the path into the dialog that appears, and click Go.
Inspecting the Sandbox
Bundle Container
This is the app’s bundle. This directory contains the app binary and all of its resources such as assets used in the app. You cannot write to this directory. To prevent tampering, the bundle directory is signed using cryptography at installation time, as mentioned before.
Data Container
It stores the data of the app for example data generated during runtime like user saving a text file, saving an image, application caches etc. It has some sub directory like:
- Document: This is a great location to store content created by users. Additionally, it’s also backed up to iCloud. The user can access the documents directory via file sharing, so it should only contain files that are appropriate for users to access.
- Library: This is used for storing application-specific data that should persist between launches. Files are deleted from the device if the device does not have enough free space. Some important purpose-specific subfolders within the library folder are as follows:
- Caches: Cache data serves to enhance application performance, though it's not essential for app. This temporary data is advantageous to persist for longer durations compared to data in the tmp directory.
- Preferences: This directory stores app-specific preference files, accessed through the NSUserDefaults API. It's crucial not to manually add files to this directory; instead, use the NSUserDefaults API. Also, the directory's contents are backed up to iCloud.
- tmp: You're able to temporarily store files required by the application. The operating system can automatically remove them when the application is not running.
Allowing user’s to access SandBox document’s
After release of iOS 11, we meet with Files app. The Files app, which allows users to manage locally stored files and files in iCloud. Additionally, this new app helps developers to allow users to access their data in app’s sandbox documents directory.
Open info.plist and add this following keys and set the boolean values as yes
-
Application supports iTunes file sharing: This property allow the user to access the files in sandbox documents directory through their Mac when they connect their device to their mac.
-
Supports opening documents in place: This property will allows the user to access files directly by using their device default file manager app without need for connecting it to a Mac.
Relaunch your app to make these changes take effect. Your files should now appear in the Files app.
In Conclusion
It's critical that you understand the purpose of the application sandbox. UIDocumentPickerViewController is an exception about modifying files or directories outside of sandbox but this is another story. Additionally, storing large files can consume a large amount of a user’s available storage, which can cause them to delete your application.