Gradients in SwiftUI
Gradients are an essential design element that should not be overlooked when designing attractive and appealing user interfaces in SwiftUI. SwiftUI Gradients offer a smooth color transition that can add depth and dimension, and a feeling of elegance to your app.
SwiftUI has four distinct gradient types: EllipticalGradient
, AngularGradient
, RadialGradient
, and LinearGradient
. A color gradient represented as an array of color stops, each having a parametric location value.
We'll explore the power of gradients in this tutorial, including how to easily integrate them into the UI design.
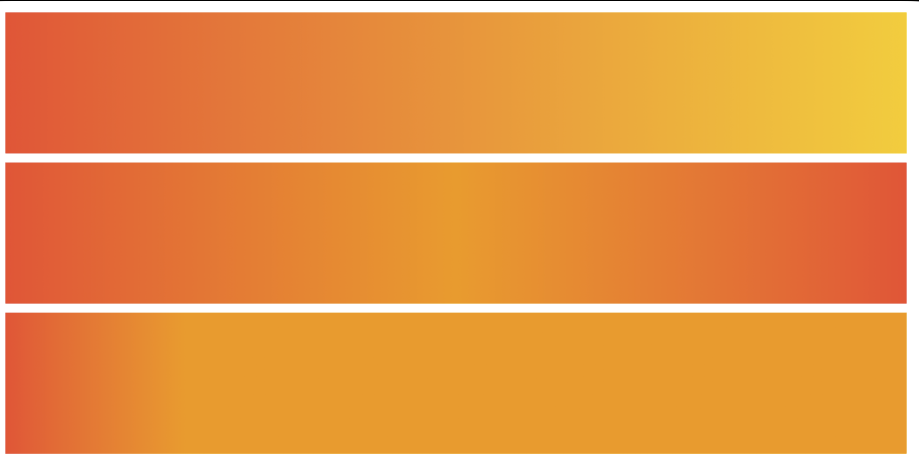
Gradient is a struct
that contains data for creating different kinds of gradients. It has two different initializer function, with Stops
and Colors
. When we initialize the gradient with Stops
, we can decide distribution of colors(in a range 0 to 1). On the other hand, Colors
object helps equally distribution.
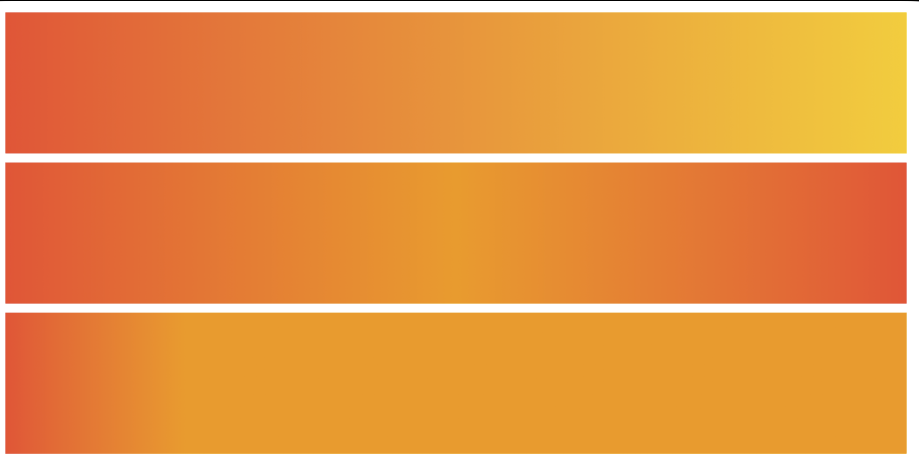
The linear gradient is the simplest gradient. You can set the beginning and ending points and a range of colors. It creates a gradient transition in a straight line between two or more colors. We can provide the positions thanks to UnitPoint
struct (x,y cordinate space). SwiftUI has predefined UnitPoint: .zero
, .top
, .bottom
, .leading
, .trailing
.
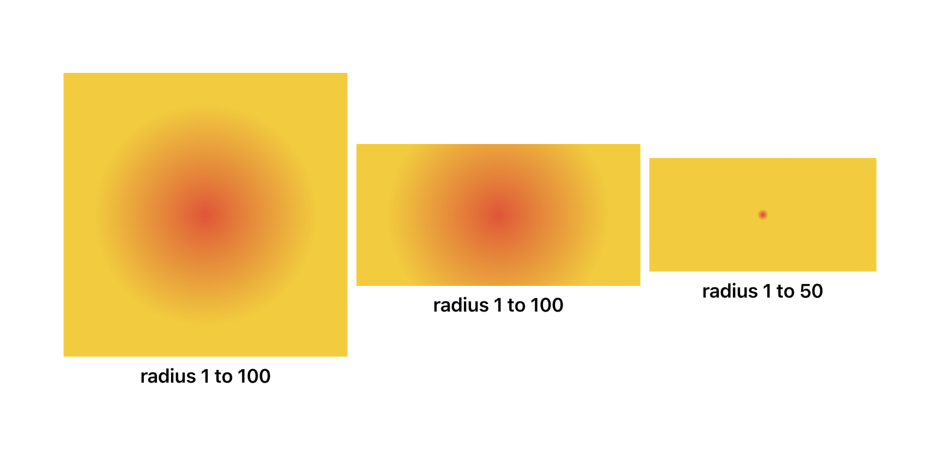
RadialGradient creates a gradient transition between two or more colors in a circular
pattern. Unlike the LinearGradient, a radial gradient begins by adding color concentrically from the center point and scales to fit within the specified radius. Concept is quite similar, we define start and end radius with center point instead of start and end points. center is UnitPoint
, startRadius and endRadius are CGFloat
objects.
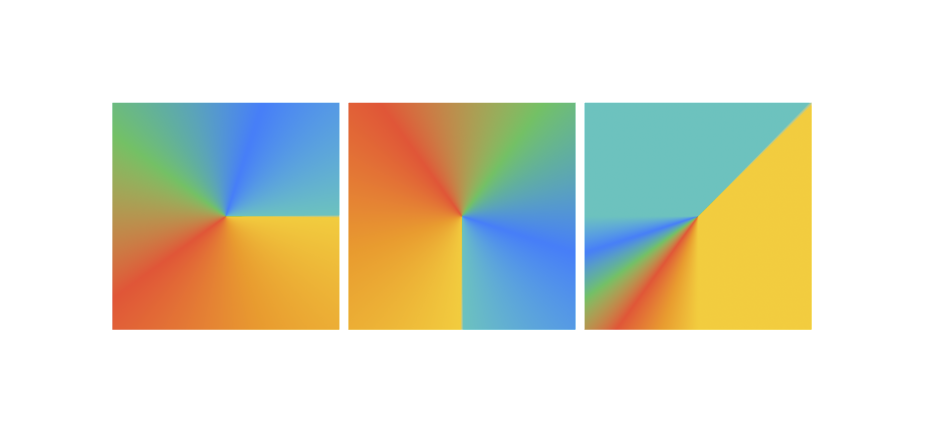
An angular gradient is also known as a conic
gradient are the gradients where the color change is based on the angle. It cycling through various colors then returning to the beginning. We can initialize AngularGradient with a few different variables.
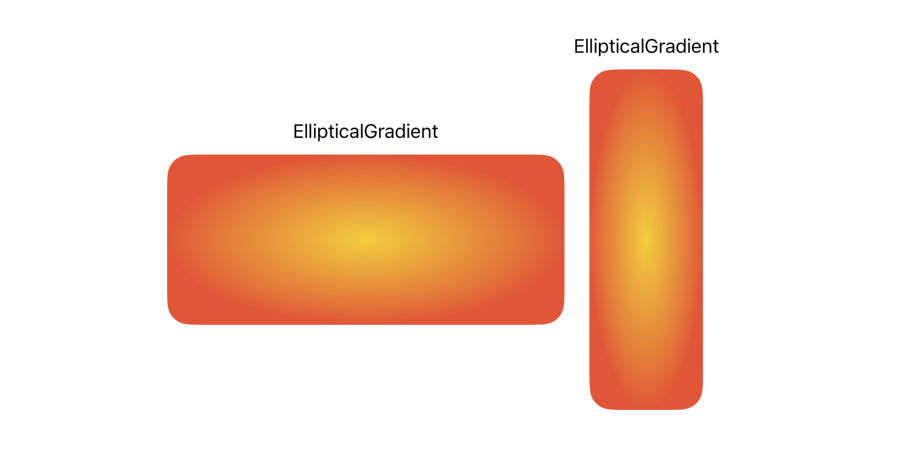
An angular gradient is also known as a “conic” gradient are the gradients where the color change is based on the angle. It cycling through various colors then returning to the beginning. We can initialize AngularGradient with a few different variables.
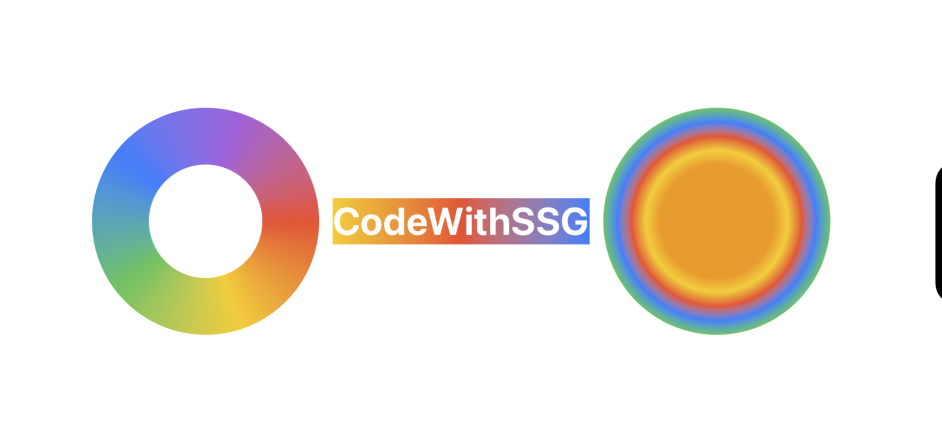
All of these gradients conform to the ShapeStyle and view protocols, therefore you can use them anywhere those two protocols will be expected. We can use them as a .background
, .stroke
and .fill
.
Gradient is a struct
that contains data for creating different kinds of gradients. It has two different initializer function, with Stops
and Colors
. When we initialize the gradient with Stops
, we can decide distribution of colors(in a range 0 to 1). On the other hand, Colors
object helps equally distribution.
The linear gradient is the simplest gradient. You can set the beginning and ending points and a range of colors. It creates a gradient transition in a straight line between two or more colors. We can provide the positions thanks to UnitPoint
struct (x,y cordinate space). SwiftUI has predefined UnitPoint: .zero
, .top
, .bottom
, .leading
, .trailing
.
RadialGradient creates a gradient transition between two or more colors in a circular
pattern. Unlike the LinearGradient, a radial gradient begins by adding color concentrically from the center point and scales to fit within the specified radius. Concept is quite similar, we define start and end radius with center point instead of start and end points. center is UnitPoint
, startRadius and endRadius are CGFloat
objects.
An angular gradient is also known as a conic
gradient are the gradients where the color change is based on the angle. It cycling through various colors then returning to the beginning. We can initialize AngularGradient with a few different variables.
An angular gradient is also known as a “conic” gradient are the gradients where the color change is based on the angle. It cycling through various colors then returning to the beginning. We can initialize AngularGradient with a few different variables.
All of these gradients conform to the ShapeStyle and view protocols, therefore you can use them anywhere those two protocols will be expected. We can use them as a .background
, .stroke
and .fill
.
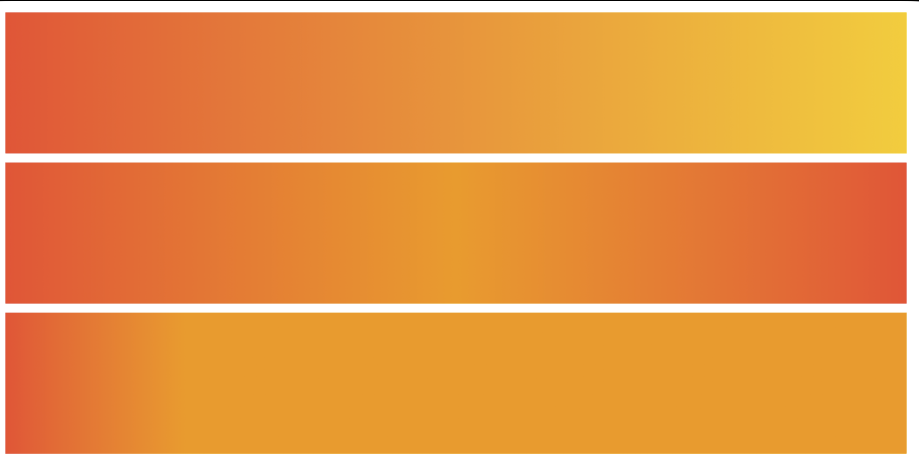